You dream to build a cross-platform GUI in Common Lisp? It’s now easy with web views.
Honestly GUIs are a difficult topic. Add in “cross platform” and you can spend your life trying out different solutions and hesitating between the best one for Common Lisp. It’s doable: Tk, Gtk3 and Gtk4, Qt4 and Qt5, CAPI (LispWorks), IUP, Nuklear, Cocoa, McCLIM, Garnet, Alloy, Java Swing… what can of worms do you want to open?
The situation improved in the last years thanks to lispers writing new bindings. So it’s possible you find one that works for your needs. That’s great, but now: you have to learn the GUI framework :p
If like me you already know the web, are developing a web app, and would like to ship a desktop application, web views are making it easy. I know the following ones, listed from least favourite to most favourite.
Table of Contents
Electron
Electron is heavy, but really cross-platform, and it has many tools around it. It allows to build releases for the three major OS from your development machine, its ecosystem has tools to handle updates, etc.
Advise: study it before discarding it.
Ceramic (old but works)
Ceramic is a set of utilities around Electron to help you build an Electron app: download the npm packages, open a browser window, etc.
Here’s its getting started snippet:
;; Start the underlying Electron process
(ceramic:start)
;; ^^^^^ this here downloads ±200MB of node packages under the hood.
;; Create a browser window
(defvar window (ceramic:make-window :url "https://www.google.com/"
:width 800
:height 600))
;; Show it
(ceramic:show window)
When you run (ceramic:bundle :ceramic-hello-world)
you get a .tar
file with your application, which you can distribute. Awesome!
But what if you don’t want to redirect to google.com but open your own
app? You just build your web app in CL, run the webserver
(Hunchentoot, Clack…) on a given port, and you’ll open
localhost:[PORT]
in Ceramic/Electron. That’s it.
Ceramic wasn’t updated in five years as of date and it downloads an
outdated version of Electron by default (see (defparameter
*electron-version* "5.0.2")
), but you can change the version yourself.
The new Neomacs project, a structural editor and web browser, is a great modern example on how to use Ceramic. Give it a look and give it a try!
What Ceramic actually does is abstracted away in the CL functions, so I think it isn’t the best to start with. We can do without it to understand the full process, here’s how.
- Ceramic API reference: http://ceramic.github.io/docs/api-reference.html
Electron from scratch
Here’s our web app embedded in Electron:

Our steps are the following:
- follow the Electron installation instructions,
- build a binary of your Lisp web app, including assets and HTML templates, if any.
- see this post: https://lisp-journey.gitlab.io/blog/lisp-for-the-web-build-standalone-binaries-foreign-libraries-templates-static-assets/ (the process will be a tad simpler without Djula templates)
- bundle this binary into the final Electron build.
- and that’s it.
You can also run the Lisp web app from sources, of course, without building a binary, but then you’ll have to ship all the lisp sources.
main.js
The most important file to an Electron app is the main.js. The one we show below does the following:
- it starts Electron
- it starts our web application on the side, as a child process, from a binary name, and a port.
- it shows our child process’ stdout and stderr
- it opens a browser window to show our app, running on localhost.
- it handles the close event.
Here’s our version.
console.log(`Hello from Electron 👋`)
const { app, BrowserWindow } = require('electron')
const { spawn } = require('child_process');
// FIXME Suppose we have our app binary at the current directory.
// FIXME This is our hard-coded binary name.
var binaryPaths = [
"./openbookstore",
];
// FIXME Define any arg required for the binary.
// This is very specific to the one I built for the example.
var binaryArgs = ["--web"];
const binaryapp = null;
const runLocalApp = () => {
"Run our binary app locally."
console.log("running our app locally…");
const binaryapp = spawn(binaryPaths[0], binaryArgs);
return binaryapp;
}
// Start an Electron window.
const createWindow = () => {
const win = new BrowserWindow({
width: 800,
height: 600,
})
// Open localhost on the app's port.
// TODO: we should read the port from an environment variable or a config file.
// FIXME hard-coded PORT number.
win.loadURL('http://localhost:4242/')
}
// Run our app.
let child = runLocalApp();
// We want to see stdout and stderr of the child process
// (to see our Lisp app output).
child.stdout.on('data', (data) => {
console.log(`stdout:\n${data}`);
});
child.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
child.on('error', (error) => {
console.error(`error: ${error.message}`);
});
// Handle Electron close events.
child.on('close', (code) => {
console.log(`openbookstore process exited with code ${code}`);
});
// Open it in Electron.
app.whenReady().then(() => {
createWindow();
// Open a window if none are open (macOS)
if (process.platform == 'darwin') {
app.on('activate', () => {
if (BrowserWindow.getAllWindows().length === 0) createWindow()
})
}
})
// On Linux and Windows, quit the app main all windows are closed.
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') {
app.quit();
}
})
Run it with npm run start
(you also have an appropriate packages.json), this gets you the previous screenshot.
JS and Electron experts, please criticize and build on it.
Missing parts
We didn’t fully finish the example: we need to automatically bundle the binary into the Electron release.
Then, if you want to communicate from the Lisp app to the Electron window, and the other way around, you’ll have to use the JavaScript layers. Ceramic might help here.
(this section was first released here: https://dev.to/vindarel/common-lisp-gui-with-electron-how-to-28fj)
What about Tauri?
Bundling an app with Tauri will, AFAIK (I just tried quickly), involve the same steps than with Electron. Tauri might still have less tools for it.
WebUI
WebUI is a new kid in town. It is in development, it has bugs. You can view it as a wrapper around a browser window (or webview.h).
However it is ligthweight, it is easy to build and we have Lisp bindings.
A few more words about it:
Use any web browser or WebView as GUI, with your preferred language in the backend and modern web technologies in the frontend, all in a lightweight portable library.
- written in pure C
- one header file
- multi-platform & multi-browser
- cross-platform webview
- we can call JS from Common Lisp, and call Common Lisp from JS.
Think of WebUI like a WebView controller, but instead of embedding the WebView controller in your program, which makes the final program big in size, and non-portable as it needs the WebView runtimes. Instead, by using WebUI, you use a tiny static/dynamic library to run any installed web browser and use it as GUI, which makes your program small, fast, and portable. All it needs is a web browser.
your program will always run on all machines, as all it needs is an installed web browser.
Sounds compelling right?
The other good news is that Common Lisp was one of the first languages it got bindings for. How it happened: I was chating in Discord, mentioned WebUI and BAM! @garlic0x1 developed bindings:
thank you so much! (@garlic0x1 has more cool projects on GitHub you can browse. He’s also a contributor to Lem)
Here’s a simple snippet:
(defpackage :webui/examples/minimal
(:use :cl :webui)
(:export :run))
(in-package :webui/examples/minimal)
(defun run ()
(let ((w (webui-new-window)))
(webui-show w "<html>Hello, world!</html>")
(webui-wait)))
I would be the happiest lisper in the world if I didn’t have an annoying issue. See #1. I can run my example just fine, but nothing happens the second time :/ I don’t know if it’s a WebUI thing, the bindings, my system, my build of WebUI… so I’ll give this more time.
Fortunately though, the third solution of this blog post is my favourite! o/
CLOG Frame (webview.h for all)
CLOG Frame is part of the CLOG framework. However, it is not tied to CLOG… nor to Common Lisp!
CLOG Frame is a short C++ program that builds an executable that takes an URL and a PORT as CLI parameters and opens a webview.h window.
It’s easy to build and works just fine.
It’s a great approach. We don’t need to develop CFFI bindings for webview.h. However such bindings would still be nice to have. I did a cursory search and didn’t find a project that seems to work. But please don’t take my word on it. Do you want to try this latest cl-webview, or have a go at the bindings?
Back to our matter.
This is CLOG Frame: 20 lines!
#include <iostream>
#include <sstream>
#include <string>
#include "webview.h"
int main(int argc,char* argv[]) {
webview::webview w(true, nullptr);
webview::webview *w2 = &w;
w.set_title(argv[1]);
w.set_size(std::stoi(argv[3]), std::stoi(argv[4]), WEBVIEW_HINT_NONE);
w.bind("clogframe_quit", [w2](std::string s) -> std::string {
w2->terminate();
return "";
});
std::ostringstream o;
o << "http://127.0.0.1:" << argv[2];
w.navigate(o.str());
w.run();
return 0;
}
Compile it on GNU/Linux like this and don’t you worry, it takes a fraction of a second:
c++ clogframe.cpp -ldl `pkg-config --cflags --libs gtk+-3.0 webkit2gtk-4.0` -o clogframe
(see its repo for other platforms)
this gives you a clogframe
binary. Put it in your $PATH or remember its location. It’s just a short C++ binary, so it weights 197Kb.
Now, back to your web app that you wrote in Common Lisp and that is waiting to be shipped to users.
Start your web app. Say it is started on port 4284.
From the Lisp side, open a CLOG Frame window like this
(uiop:launch-program (list "./clogframe"
"Admin"
(format nil "~A/admin/" 4284)
;; window dimensions (strings)
"1280" "840"))
and voilà.
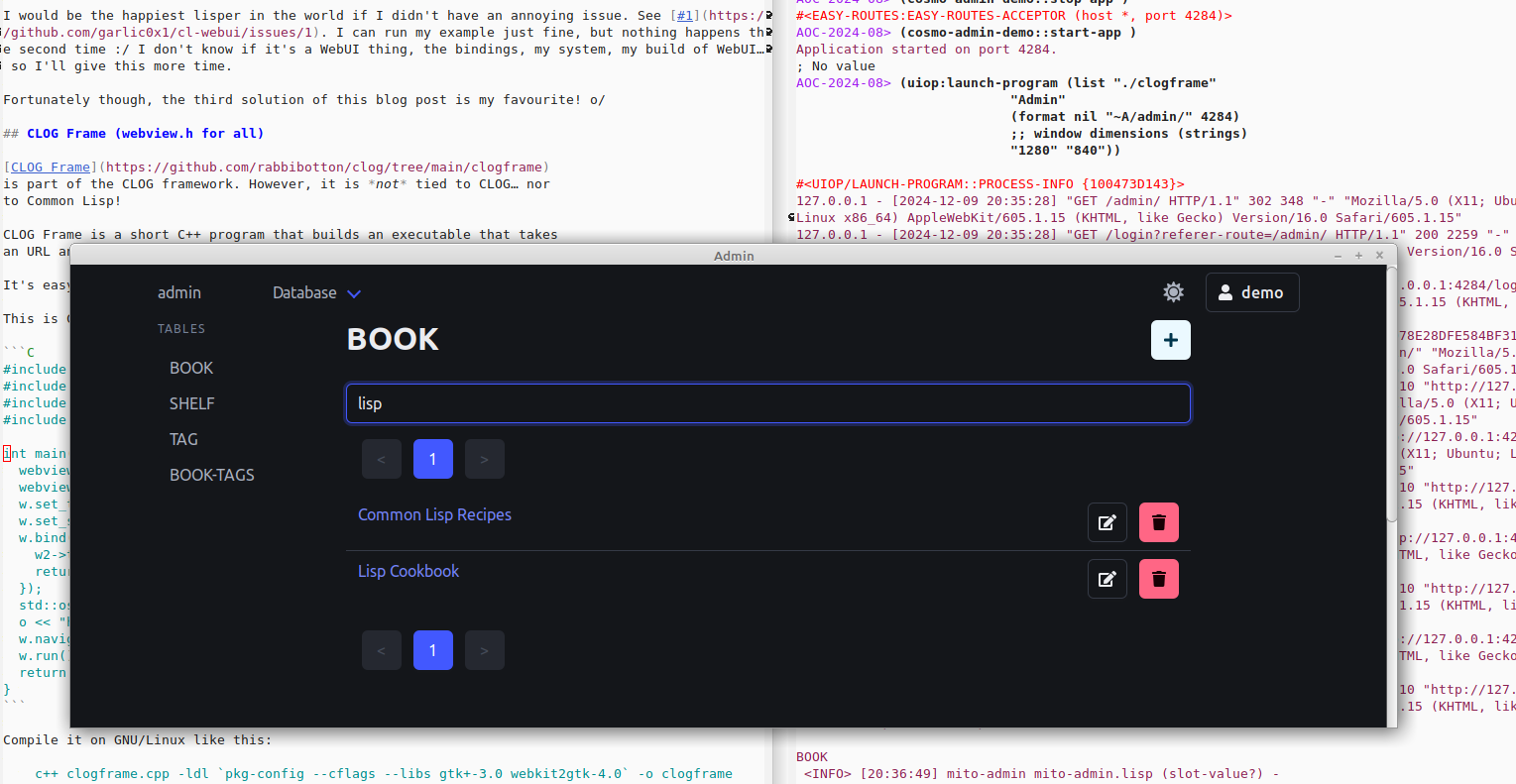
Now for the cross-platform part, you’ll need to build clogframe and your web app on the target OS (like with any CL app). Webview.h is cross-platform. Leave us a comment when you have a good CI setup for the three main OSes (I am studying 40ants/ci and make-common-lisp-program for now).
CLOG Frame should be a (popular) project on its own IMO! (@dbotton might make it independant eventually)
Please share any experience you might have on the topic 👍