Last week I finished a new service written in Common Lisp. It now runs in production© every mornings, and it expands the set of services I offer to clients.
It’s the 4th service of this kind that I developed: - they are not big - but have to be done nonetheless, and the quicker the better (they each amount to 1k to 2k lines of Lisp code), - they are not part of a super advanced domain that requires Common Lisp superpowers - I am the one who benefits from CL during development, - I could have written them in Python - and conversely nothing prevented me from writing them in Common Lisp.
So here lies the goal of this post: illustrate that you don’t need to need a super difficult problem to use Common Lisp. This has been asked many times, directly to me or on social media :)
At the same time, I want to encourage you to write a little something about how you use Common Lisp in the real world. Sharing creates emulation. Do it! If you don’t have a blog you can simply write in a new GitHub repository or in a Gist and come share on /r/lisp. We don’t care. Thanks <3
We’ll briefly see what my scripts do, what libraries I use, how I deploy them, what I did along the way.
Needless to say that I dogfooded my CIEL (beta) meta-library and scripting tool for all those projects.
Table of Contents
- Scripts n°4 and 2 - shaping and sending data - when you can write Lisp on the side
- Scripts n°3 and 1 - complementary web apps
- Lasting words
- Links
Scripts n°4 and 2 - shaping and sending data - when you can write Lisp on the side
My latest script needs to read data from a DB, format what’s necessary according to specifications, and send the result by SFTP.
In this case I read a DB that I own, created by a software that I develop and host. So I could have developed this script in the software itself, right? I could have, but I would have been tied to the main project’s versioning scheme, quirks, and deployment. I rather had to write this script on the side. And since it can be done on the side, it can be done in Common Lisp.
I have to extract products and their data (price, VAT…), aggregate the numbers for each day, write this to a file, according to a specification.
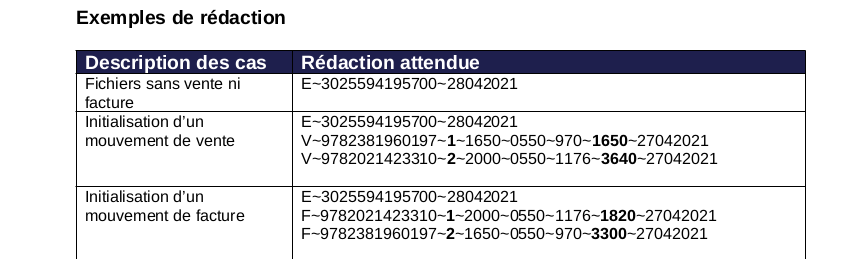
To read the DB, I used cl-dbi
. I didn’t format the SQL with SxQL
this time like in my web apps (where I use the Mito light ORM), but I wrote SQL directly. I’m spoiled by the Django ORM
(which has its idiosyncrasies and shortcomings), so I double checked
the different kinds of JOINs and all went well.
I had to group rows by some properties, so it was a great time to use serapeum:assort
. I left you an example here: https://dev.to/vindarel/common-lisps-group-by-is-serapeumassort-32ma
Dates have to be handled in different formats. I used local-time
of
course, and I still greatly appreciate its lispy formatter syntax:
(defun date-yymmddhhnnss (&optional date stream)
(local-time:format-timestring stream
(or date (local-time:now))
:format
'((:year 4)
(:month 2)
(:day 2)
(:hour 2)
(:min 2)
(:sec 2)
)))
the 2 in (:month 2)
is to ensure the month is written with 2 digits.
Once the file is written, I have to send it to a SFTP server, with the client’s codes.
I wrote a profile
class to encapsulate the client’s data as well as
some functions to read the credentials from either environment
variables, the file system, or a lisp variable. I had a top-level
profile object for ease of testing, but I made sure that my functions
formatting or sending data required a profile
parameter.
(defun send-stock (profile &key date) …)
(defun write-stock (profile filename) …)
Still nothing surprising, but it’s tempting to only use global parameters for a one-off script. Except the program grows and you pay the mess later.
SFTP
To send the result through SFTP, I had to make a choice. The SFTP
command line doesn’t make it possible to give a password as argument
(or via an environment variable, etc). So I use lftp
(in Debian
repositories) that allows to do that. In the end, we format a command
like this:
lftp sftp://user:****@host -e "CD I/; put local-file.name; bye"
You can format the command string and run it with uiop:run-program
:
no problem, but I took the opportunity to release another utility:
First, you create a profile
object. This one-liner reads the
credentials from a lispy file:
(defvar profile (make-profile-from-plist (uiop:read-file-form "CREDS.lisp-expr"))
then you define the commands you’ll want to run:
(defvar command (put :cd "I/" :local-filename "data.csv"))
;; #<PUT cd: "I/", filename: "data.csv" {1007153883}>
and finally you call the run
method on a profile and a command. Tada.
Deploying
Build a binary the classic way (it’s all on the Cookbook), send it to your server, run it.
(during a testing phase I have deployed “as a script”, from sources, which is a bit quicker to pull changes and try again on the server)
Set up a CRON job.
No Python virtual env to activate in the CRON environment…
Add command line arguments the easy way or with the library of your choice (I like Clingon).
Script n°2 and simple FTP
My script #2 at the time was similar and simpler. I extract the same products but only take their quantities, and I assemble lines like
EXTRACTION STOCK DU 11/04/2008
....978202019116600010000001387
....978270730656200040000000991
For this service, we have to send the file to a simple FTP server.
We have a pure Lisp library for FTP (and not SFTP) which works very well, cl-ftp.
It’s a typical example of an old library that didn’t receive any update in years and so that looks abandoned, that has seldom documentation but whose usage is easy to infer, and that does its job as requested.
For example we do this to send a file:
(ftp:with-ftp-connection (conn :hostname hostname
:username username
:password password
:passive-ftp-p t)
(ftp:store-file conn local-filename filename))
I left you notes about cl-ftp and my SFTP wrapper here:
Scripts n°3 and n°1 - specialized web apps
A recent web app that I’m testing with a couple clients extends an existing stock management system.
This one also was done in order to avoid a Python monolith. I still needed additions in the Python main software, but this little app can be independent and grow on its own. The app maintains its state and communicates it with a REST API.
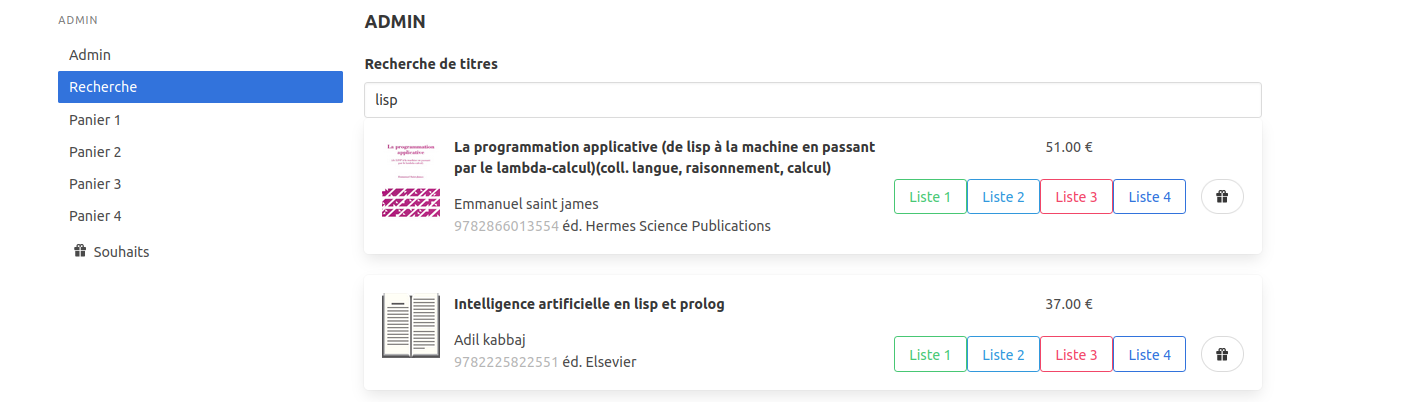
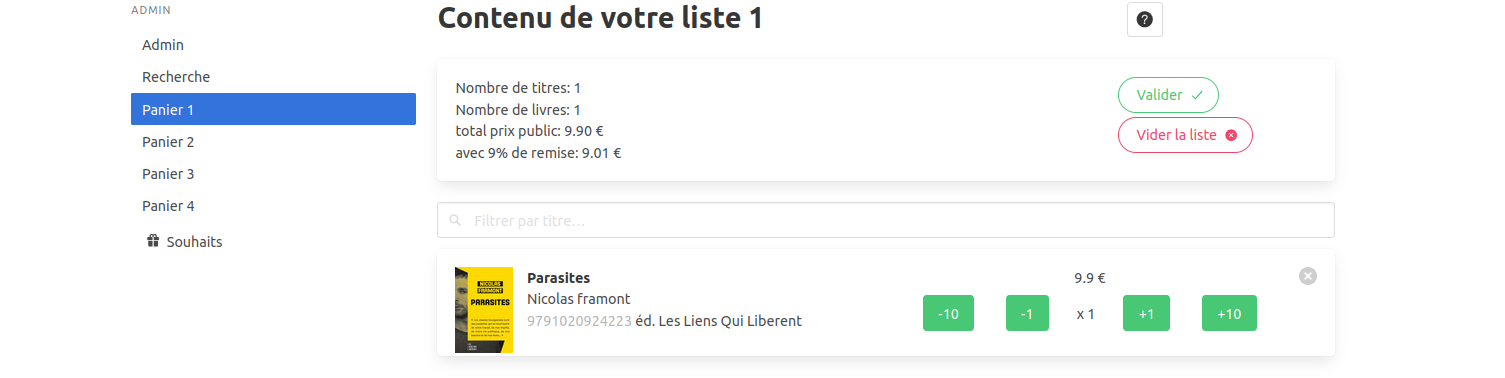
It gives a web interface to their clients (so my clients’ clients, but not all of them, only the institutional) so that they can:
- search for products
- add them in shopping carts
- validate the cart, which sends the data to the main software and notifies the owner, who will work on them.
The peculiarities of this app are that:
- there is no user login, we use unique URLs with UUIDs in the form:
http://command.client.com/admin-E9DFOO82-R2D2-007/list?id=1
- I need a bit of file persistence but I didn’t want the rigidity of a database so I am using the clache library. Here also, not a great activity, but it works©. I persist lists and hash-tables. Now that the needs grow and the original scope doesn’t cut it any more, I wonder how long I’ll survive without a DB. Only for its short SQL queries VS lisp code to filter data.
I deploy a self-contained binary: code + html templates in the same binary (+ the implementation, the web server, the debugger…), with Systemd.
I wrote more on how to ship a standalone binary with templates and static assets with Djula templates here:
I can connect to the running app with a Swank server to check and set parameters, which is super helpful and harmless.
It is possible to reload the whole app from within itself and I did it with no hiccups for a couple years, but it isn’t necessary the most reliable, easiest to set up and fastest method. You can do it, but nobody forces you to do this because you are running CL in production. You can use the industry’s boring and best practices too. Common Lisp doesn’t inforce a “big ball of mud” approach. Develop locally, use Git, use a CI, deploy a binary…
Every thing that I learned I documented it along the way in the Cookbook ;)
Another app that I’ll mention but about which I also wrote earlier is my first web app. This one is open-source. It still runs :)
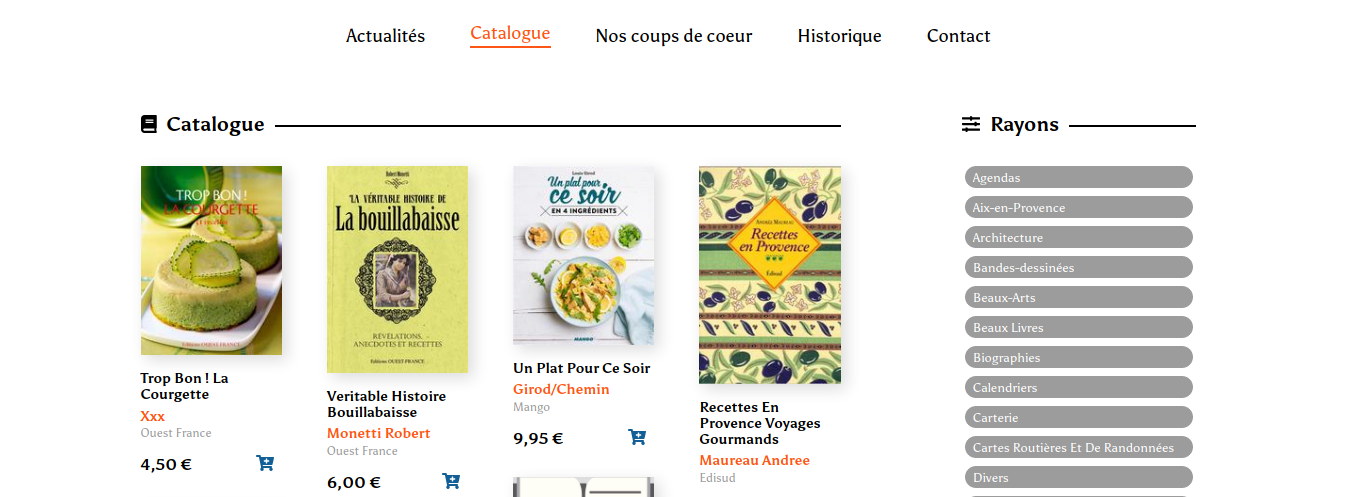
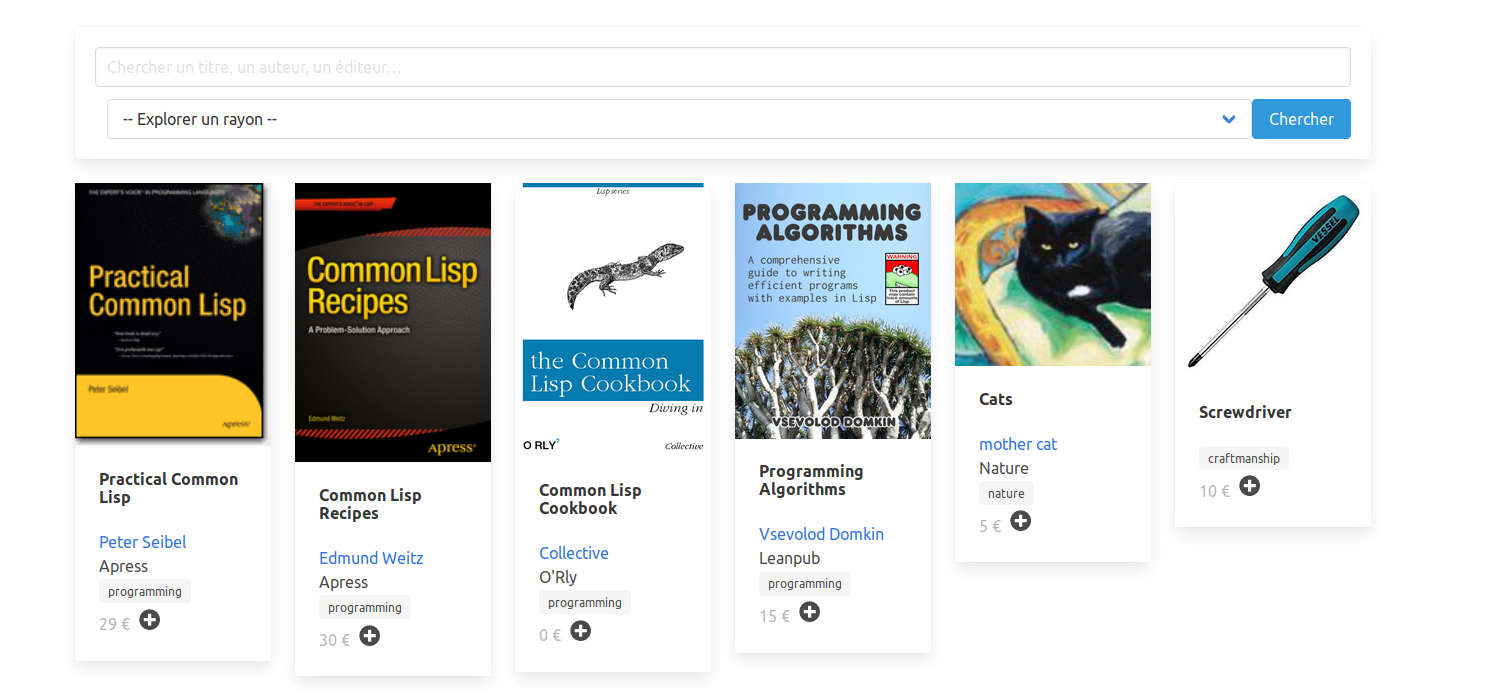
In this project I had my friend and colleague contribute five lines of Lisp code to add a theme switcher in the backend that would help him do the frontend. He had never written a line of Lisp before. Of course, he did so by looking at my existing code to learn the existing functions at hand, and he could do it because the project was easy to install and run.
(defun get-template(template &optional (theme *theme*))
"Loads template from the base templates directory or from the given theme templates directory if it exists."
(if (and (str:non-blank-string-p theme)
(probe-file (asdf:system-relative-pathname "abstock" (str:concat "src/templates/themes/" theme "/" template))))
;; then
(str:concat "themes/" theme "/" template)
;; else :D
template))
He had to annotate the if
branches :] This passed the code review.
Lasting words
The 5th script/app is already on the way, and the next ones are awaiting that I open their .docx specification files. This one was a bit harder but the Lisp side was done sucessfully with the efficient collaboration of another freelance lisper (Kevin to not name him).
All those tasks (read a DB, transform data…) are very mundane.
They are everywhere. They don’t always need supercharged web framework or integrations.
You have plenty of opportunities to make yourself a favor, and use Common Lisp in the wild. Not counting the super-advanced domains where Lisp excels at ;)
Links
- https://lispcookbook.github.io/cl-cookbook/
- awesome-cl
- companies using Common Lisp in production (at least the ones we know)
- Common Lisp course in videos – it helps me, and you ;) I added 9 videos about CLOS last month, and more are coming. It’s 86 minutes of an efficient code-first approach, out of 7+ hours of total content in the course. After this chapter you know enough to read the sources of the Hunchentoot web server or of the Kandria game.
I have done some preliminary Common Lisp exploration prior to this course but had a lot of questions regarding practical use and development workflows. This course was amazing for this! I learned a lot of useful techniques for actually writing the code in Emacs, as well as conversational explanations of concepts that had previously confused me in text-heavy resources. Please keep up the good work and continue with this line of topics, it is well worth the price! [Preston, October of 2024]